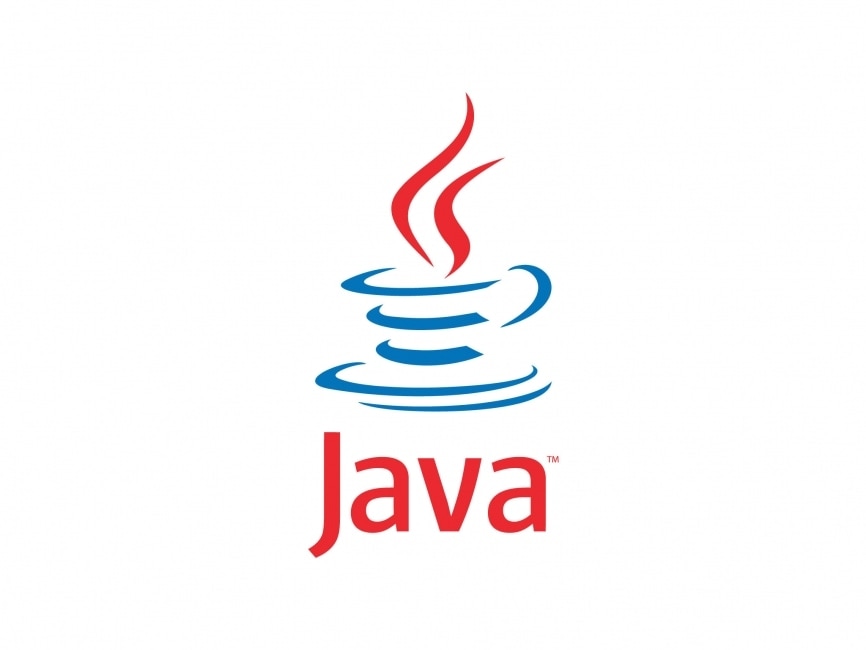
package practice;
import java.util.Scanner;
public class StudentAnalyze {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
boolean runFlag = true;
int studentNum = 0;
int[] scores = null;
while (runFlag) {
System.out.println("------------------------------------------");
System.out.println("1.학생 수 | 2.점수입력 | 3.점수리스트 | 4. 분석 | 5. 종료");
System.out.println("------------------------------------------");
System.out.print("선택> ");
int menuChoice = scanner.nextInt();
switch (menuChoice) {
case 1: {
System.out.print("학생 수> ");
studentNum = scanner.nextInt();
scores = new int[studentNum];
break;
}
case 2: {
if (studentNum == 0) {
System.out.println("학생 수부터 입력해주세요.");
break;
} else {
for (int inputScore = 0; inputScore < studentNum; inputScore++) {
System.out.print((inputScore + 1) + ("번째 학생의 점수: "));
scores[inputScore] = scanner.nextInt();
}
break;
}
}
case 3: {
if (studentNum == 0) {
System.out.println("학생 수부터 입력해주세요.");
break;
} else {
for (int printScore = 0; printScore < studentNum; printScore++) {
System.out.println((printScore + 1) + ("번째 학생의 점수: ") + scores[printScore]);
}
}
break;
}
case 4: {
int max = 0;
int sum = 0;
double avg = 0;
if (studentNum == 0) {
System.out.println("학생 수부터 입력해주세요.");
break;
} else {
for (int entireScore = 0; entireScore < studentNum; entireScore++) {
if (scores[entireScore] > max) {
max = scores[entireScore];
}
sum += scores[entireScore];
}
avg = (double) sum / studentNum;
System.out.println("최고 점수: " + max);
System.out.println("점수 평균: " + avg);
break;
}
}
case 5: {
runFlag = false;
System.out.println("프로그램 종료");
break;
}
default: {
System.out.println("번호를 잘못 입력했습니다. 다시 입력해주세요.");
break;
}
}
}
}
}
오늘의 배운 점
다른 건 다 되는데 4번 분석에서 1시간 걸렸다. 로직 자체는 맞는데 왜 최고 점수랑 평균이 안 맞는지 의아했다.
알고보니 스코프 문제가 발생해서 그런거였다..ㅎㅎ.. `sum += scores[entireScore];`를 `max = scores[entireScore];` 밑에 뒀더니 당연히 총합이 안 나올 수 밖에 없었다. 평균 구하는 것도 영역을 이상한데에 둬서 그런거였다.
어쩐지 85, 95, 93이 주어졌는데 85가 나오더라.
1. 디버깅을 적극적으로 사용하자
2. 뭔가 값이 이상하게 나온다면 스코프를 확인하자.
출처
1. 이것이 자바다 200p 연습문제 9번
'TIL > Java' 카테고리의 다른 글
[TIL/Java] 객체 동등 비교 equals() (0) | 2024.08.21 |
---|---|
[TIL/Java] 다형성, 필드 다형성, 추상 클래스 (abstract) (0) | 2024.08.19 |
[TIL/Java] 배열 항목에서 최대값 출력하기 (for문 이용) (0) | 2024.08.12 |
[TIL/Java] 컴파일러란? (0) | 2024.08.07 |
[TIL/Java] JPA - ddl-auto 주의점 (0) | 2023.11.14 |