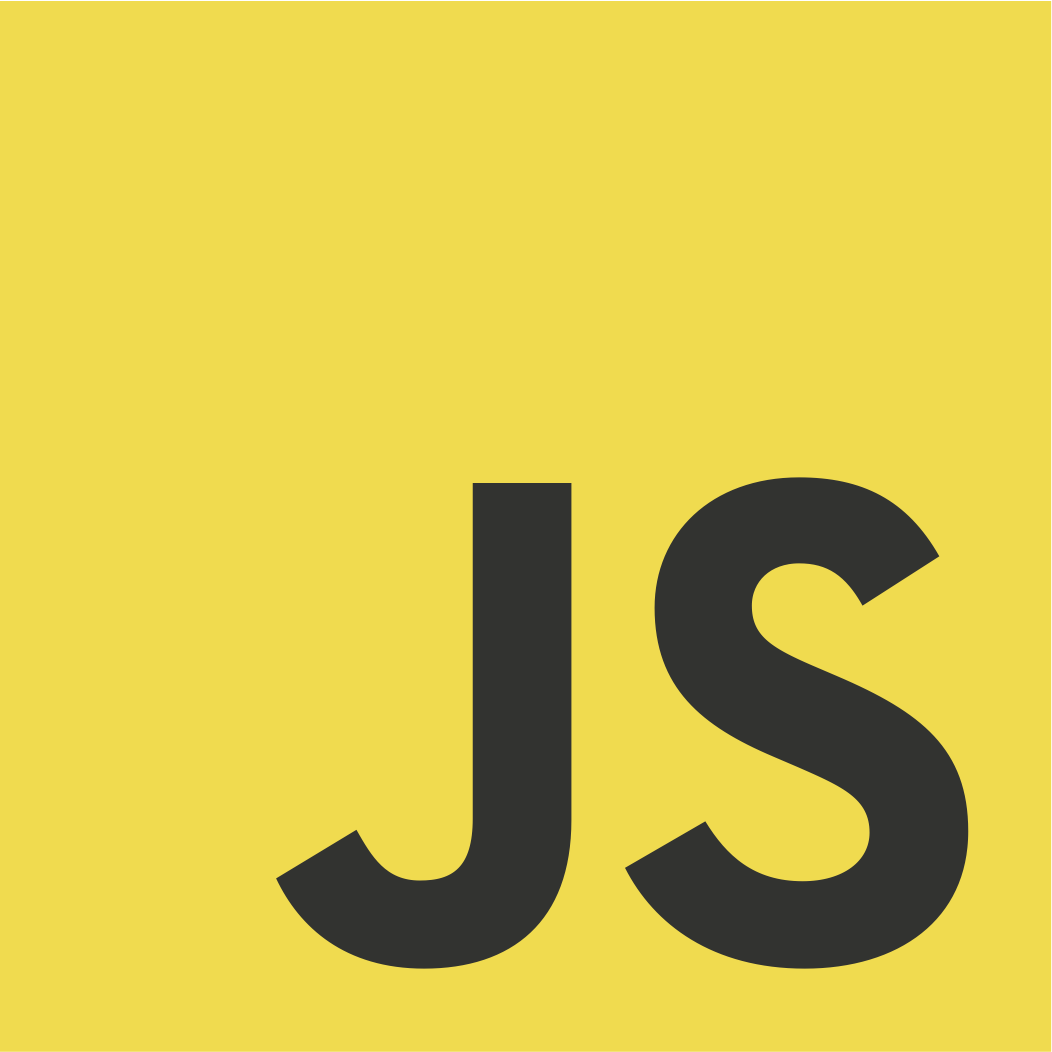
Published 2024. 7. 2. 23:07
파라미터 이름과 클래스 필드 이름 일치
생성자서 받는 파라미터 이름과 클래스 필드 이름이 일치해야 한다.
자바스크립트가 변수와 속성 이름을 정확히 구분하기 때문에 이름이 일치하지 않으면 원하는 값이 제대로 할당되지 않기 때문이다.
class Picture
부분과 document.write
부분을 보면 파라미터와 클래스 필드가 매칭된다.
class Picture {
constructor(aTitle, aImgAddr, aAuthor, aYear) {
this.aTitle = aTitle;
this.aImgAddr = aImgAddr;
this.aAuthor = aAuthor;
this.aYear = aYear;
}
show() {
document.write(
`<dl><dt>${this.aTitle}</dt><dd><img src="${this.aImgAddr}"></dd><dd>${this.aAuthor} ${this.aYear}</dd></dl>`
);
}
}
let arrPicture = []; // 배열 생성
// 배열에 Picture 삽입
arrPicture.push(new Picture('에펠탑의 신랑신부', './images/pic01.jpg', '샤갈', 1864));
arrPicture.push(new Picture('꿈', './images/pic02.jpg', '피카소', 1865));
arrPicture.push(new Picture('무용실', './images/pic03.jpg', '드가', 1866));
arrPicture.push(new Picture('피아노 치는 소녀들', './images/pic04.jpg', '르누아르', 1867));
// for문 조건식 -i 가 배열의 길이보다 작을 때까지 반복
for (let i = 0; i < arrPicture.length; i++) {
arrPicture[i].show();
}
만약 필드 이름을 다르게 하고 싶다면 생성자에서 그 필드에 올바르게 할당하면 된다.
class Picture {
constructor(aTitle, aImgAddr, aAuthor, aYear) {
this.title = aTitle;
this.imgAddr = aImgAddr;
this.author = aAuthor;
this.year = aYear;
}
show() {
document.write(
`<dl><dt>${this.title}</dt><dd><img src="${this.imgAddr}"></dd><dd>${this.author} ${this.year}</dd></dl>`
);
}
}
this 키워드
자바스크립트에서 클래스 내부의 `this`는 생성된 객체를 가리킨다.
따라서 `this.title`은 클래스 인스턴스의 속성을 의미한다.
class Picture {
constructor(title) {
this.title = title;
}
위와 같은 구문이 있다면 오른쪽의 `title`은 생성자 매개변수로 전달된 값을 의미한다.
왼쪽의 `this.title`은 새로운 `Picture` 객체의 속성을 설정한다.
클래스 내부에서 따로 속성을 선언하지 않아도 `this` 키워드를 이용하면 매개변수로 받은 값을 객체의 속성으로 선언하고 할당할 수 있다.
class Picture {
constructor(title, imgAddr, author, year) {
this.title = title;
this.imgAddr = imgAddr;
this.author = author;
this.year = year;
}
1. 매개변수로 받은 값을 객체의 속성으로 할당하기
- `constructor(title, imgAddr, author, year)`는 `title`, `imgAddr`, `author`, `year` 값을 매개변수로 받는다.
- `this.title = title;`은 매개변수로 받은 `title` 값을 객체의 `title` 속성으로 할당한다.
2. `this` 키워드의 역할
- `this` 키워드는 현재 인스턴스를 가리킨다.
- 예를 들어, 'new Picture('에펠탑의 신랑신부', './images/pic01.jpg', '샤갈', 1864)`를 호출하면 `this`는 새로 생성된 `Picture` 객체를 가리킨다.
constructor()
생성자 함수인 `contructor()`, 객체가 생성될 때 호출되며, 객체를 초기화 하는 역할을 한다.
constructor(title, imgAddr, author, year)
새로운 `Picture` 객체가 생성될 때 호출되고, 객체의 title`, `imgAddr`, `author`, `year` 속성을 초기화 한다.
'TIL > JavaScript' 카테고리의 다른 글
[TIL/JS] Number 보다 parseInt()를 더 권고하는 이유 (0) | 2024.09.06 |
---|---|
[TIL/JavaScript] 홀수 인덱스의 요소와 홀수번째 요소 (0) | 2024.07.15 |
[TIL/JavaScript] 갤러리 만들기 (0) | 2024.07.03 |
[TIL/JavaScript] 후위 연산자와 리팩토링 (0) | 2024.07.03 |
[TIL/JavaScript] script 태그, 이벤트, 문자열과 숫자 (0) | 2023.11.20 |